프로그래밍/코딩테스트
[LeetCode] 21. Merge Two Sorted Lists / Swift
turu
2021. 6. 20. 19:54
[문제 보기]
더보기
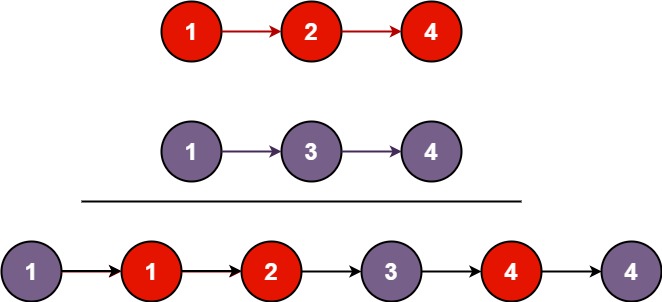
Merge two sorted linked lists and return it as a sorted list. The list should be made by splicing together the nodes of the first two lists.
Example 1:
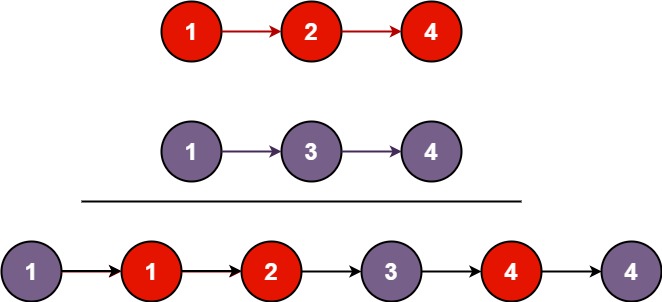
Input: l1 = [1,2,4], l2 = [1,3,4] Output: [1,1,2,3,4,4]
Example 2:
Input: l1 = [], l2 = [] Output: []
Example 3:
Input: l1 = [], l2 = [0] Output: [0]
Constraints:
- The number of nodes in both lists is in the range [0, 50].
- -100 <= Node.val <= 100
- Both l1 and l2 are sorted in non-decreasing order.
https://leetcode.com/problems/merge-two-sorted-lists/
Merge Two Sorted Lists - LeetCode
Level up your coding skills and quickly land a job. This is the best place to expand your knowledge and get prepared for your next interview.
leetcode.com
간단한 연결리스트 문제였다.
/**
* Definition for singly-linked list.
* public class ListNode {
* public var val: Int
* public var next: ListNode?
* public init() { self.val = 0; self.next = nil; }
* public init(_ val: Int) { self.val = val; self.next = nil; }
* public init(_ val: Int, _ next: ListNode?) { self.val = val; self.next = next; }
* }
*/
class Solution {
func mergeTwoLists(_ l1: ListNode?, _ l2: ListNode?) -> ListNode? {
var p1 = l1
var p2 = l2
var ans = ListNode()
let first = ans
while p1 != nil || p2 != nil {
if let v1 = p1?.val, let v2 = p2?.val {
if v1 < v2 {
ans.next = ListNode(v1)
ans = ans.next!
p1 = p1!.next
} else {
ans.next = ListNode(v2)
ans = ans.next!
p2 = p2!.next
}
} else {
var temp = p1
if temp == nil {
temp = p2
}
while temp != nil {
ans.next = ListNode(temp!.val)
ans = ans.next!
temp = temp!.next
}
break
}
}
// var temp: ListNode? = first.next
// while temp != nil {
// print(temp!.val)
// temp = temp!.next
// }
return first.next
}
}
반응형